Working with Mandrill in Django
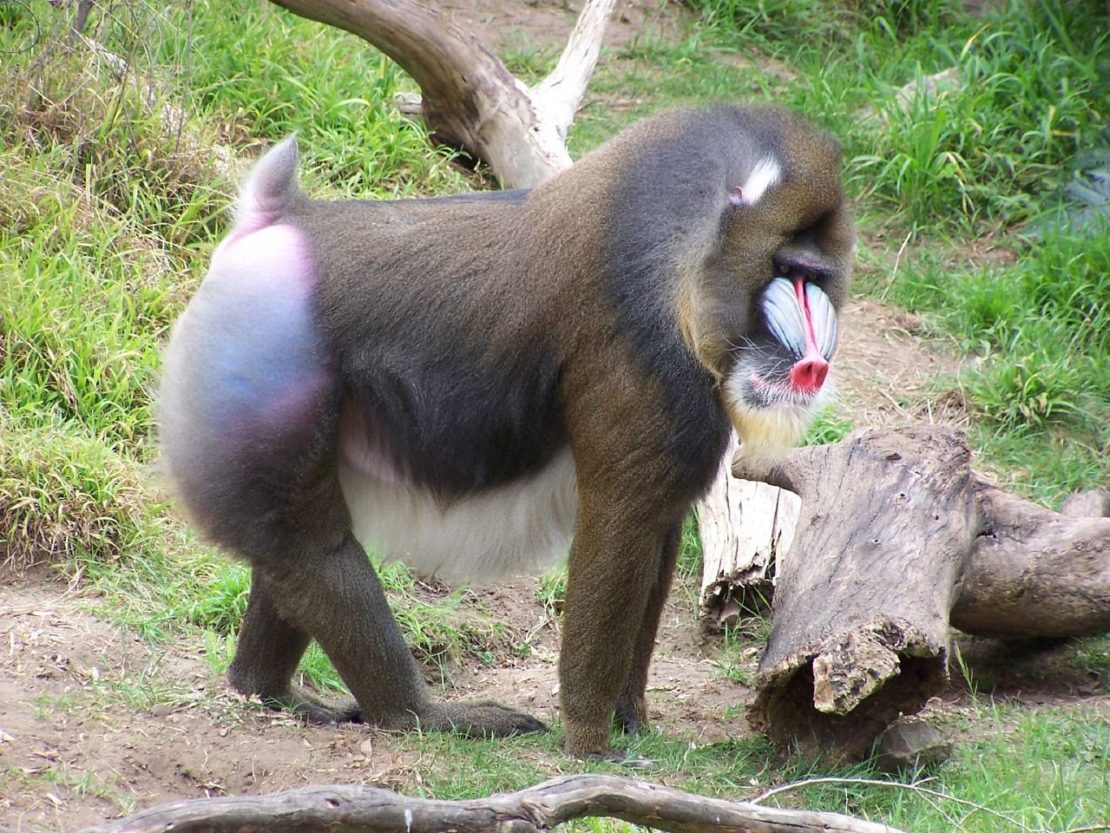
One of the most tedious things for me as a developer is updating website content at a client’s request. Updating content is something that the client should be able to do themselves, but it is our duty as developers to give them a tool to do so. Let’s take emails as an example. Clients need to update the content of the emails they send out from time to time.
Mandrill
Sending emails directly from Django means that you need to keep all your email templates on the server, and it’s time consuming to create a tool that gives clients access to update these templates. This is where Mandrill gives us a hand. Mandrill is “a scalable and affordable email infrastructure service, with all the marketing-friendly analytics tools you’ve come to expect from MailChimp.” Most importantly for us, Mandrill keeps our templates and gives us an API to be able to use them for sending emails by passing only email context.
Django + Mandrill = Djrill
Djrill integrates Mandrill’s transactional email service into Django. Here are the 4 steps to set it up in your Django project:
pip install djrill
- Add djrill to
INSTALLED_APPS
- Define api key in django settings:
MANDRILL_API_KEY = "mandrill-api-key"
- Define email backend
EMAIL_BACKEND = "djrill.mail.backends.djrill.DjrillBackend"
Sending emails from Django using Mandrill template
First, let’s define the email template in Mandrill. We’ll name it “test email” (slug: “test-email”)
Hi *|FOO|*, here is a link to our website *|URL|*
Now, our first email is ready to be sent:
from django.core.mail import EmailMessage msg = EmailMessage(to=["foo@bar"]) msg.template_name = "test-email" # slug from Mandrill msg.global_merge_vars = { 'foo': "bar", 'url': "tivix.com" } msg.use_template_subject = True msg.use_template_from = True msg.send()
And that’s it!