What’s so cool about D3?
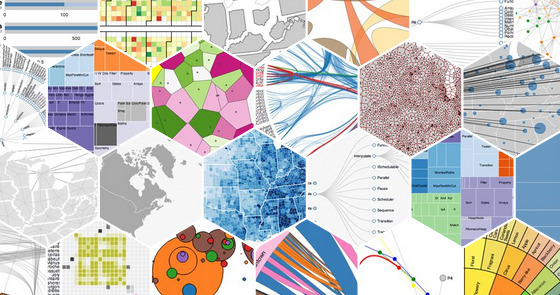
In the past couple years, the web has seen a change in how data is presented to the world. For example, this interactive Poverty Map from The New York Times. Marked by Hans Rosling’s TED talk in 2007, showcasing his tool called Gapminder, interactive tools started to evolve. Since then, the technology behind it has grown into thousands of libraries and modules, the main one being D3.js. This JavaScript library has made it immensely easier to deal with data and incorporate them on any web page.
D3 stands for Data Driven Documents, which is a good way to think about how it works. Most d3.js files have an array of data, which is mapped to (or drives) a tag on the DOM. The success of D3 can be attributed to the flexibility and wide range of its use. You can even plug it into any web page in a browser’s developer tools and start manipulating things on the fly, just to play around with it. The creator of D3, Mike Bostock, has also done an incredible job making helpful content such as tutorials, examples, and source code.
It wasn’t until last semester, while working in a lab at Georgia Tech, that I was introduced to this field. For me, I had found the perfect combination of my interests — design and code — and I wanted to share a couple nifty things about this library with you all.
Selections
You can pass in selectors to either d3.select( ) or d3.selectAll( ), which works a lot like jQuery. This is what allows D3 to be so integrated with the DOM. Something to note is that these will return a reference to the DOM as an array of arrays. This means that a single element returns a nested array. For example, selecting d3.select(‘#id’), will return [[id’s element]]. The way this is set up, it is easier to grab subsections of the DOM and manipulate them as a group.
Chainability
There are a couple features that will make your code a lot smaller. One is chainability. Although this concept isn’t new, it plays a key role in D3 code. When looking at examples, you will notice that the code usually consists of long pipes of functions. This is possible because most functions return the selection that was just modified.
Enter and Exit
One concept that can be hard to grasp is the enter( ) method. This is used when you need to append new objects to the DOM that aren’t there yet. For example, if you only have two header elements, but the array it’s bound to has four values, enter( ).append( ) will allow it to add two more headers elements to the DOM. On the other hand, the exit( ) method will allow you to remove elements when there are too many.
These two functions are the key to calling the D3 library “dynamic.” They allow for easy manipulation of elements by group when creating an animation, which is especially useful when dealing with thousands of data points. You can dynamically create SVG elements as you go, or have them bounce off the screen when needed, all in one command.
Scales
A particularly handy feature of D3 is the ability to scale a graphic. For example, if you want to change the dimensions of your graph from a 200 pixel area to a 300 pixel area, this is a tedious linear translation of every point on the graph. D3 will handle the math. To scale an element, use d3.scale, and then select the type you’d like. They provide linear, exponential and logarithmic scales.
var scale = d3.scale.linear();
After the type of scale is selected, you can use .domain( ) and .range( ) to set your input and output. The following line will take in values with a min of 0 and a max of 200, and scale it to an area of 0 to 300 pixels.
var scale = d3.scale.linear().domain([0, 200]).range([0, 300]);
One thing to note is that in SVG, the y-axis is upside down from the conventional graph. So, the origin (0,0) is in the top left corner of the screen.
Animation
Animations used to be one of the trickiest parts of any visual project. D3’s .transition() handles this in a seamless manner. For example:
d3.select("div").transition().duration(1000).style("opacity",0);
This will select whatever is in the div, and take 1,000 milliseconds to make the opacity 0. The possibilities within transition are endless. This blog post on Visual.ly is a great place to checkout some other features.