Type Hints in Python 3
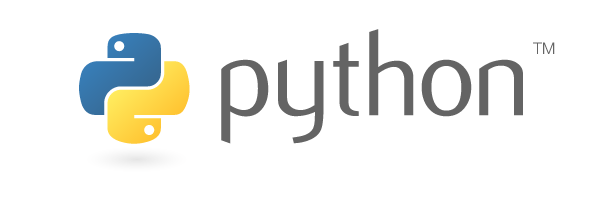
Among the new features in Python 3, there’s an interesting syntax addition — namely, function annotation syntax. It allows adding annotations to function parameters and their return values. It can serve as a way of documenting a function, for example, by using string descriptions like this:
def test_view(view: "view object to test", attrs: "additional attributes, such as `skip_session_check`, etc." = None): ...
Or by indicating the type:
def to_date(date_string: str) -> datetime: return datetime.strptime(date_string,'%Y-%m-%d')
This syntax provides opportunity to document the function as well as allows third party tools to provide static analysis, but without any specific rules or strictly defined standards on how to perform such analysis. PEP 484, implemented in Python 3.5.0, introduces semantics and standard vocabulary for using function annotations specifically for type checking. The proposal is based on mypy. It includes a typings module which contains tools and definitions for type hints, and allows more complex types such as generics:
from typing import List, Dict def stringify_list(numbers: List[int]) -> List[str]: return [str(number) for number in numbers] def print_data(attrs: Dict[int, str]) -> None: for key, value in attrs.items(): print('{0}: {1}'.format(key, value))
It also has special type Any
that is compatible with any type:
from typing import Any, Dict def get(request: HttpRequest, attrs: Dict[str, Any]) -> HttpResponse: ... return response
More typing module examples can be found in Python Docs.
Type hints can be retrieved with typings.get_type_hints(function_name)
. The decorator @no_type_check
or adding # type: ignore
can be used to indicate that type hints should be ignored.
Although the module provides structure for specifying type hints, there’s no type checking in core Python involved. As the PEP 484 states,
Python will remain a dynamically typed language, and the authors have no desire to ever make type hints mandatory, even by convention.
What this addition provides is a standard syntax for static analysis in Python that can be used by third party tools.
There are currently existing implementations that use this syntax to provide static type checking for Python. For example, it is implemented in the PyCharm IDE, where it will display a warning if the parameter of wrong type is passed into the function:
Type checking provides a lot of benefits for the development process. It makes code more robust and it allows catching certain types of bugs early in the process. It also provides additional documentation for the code and makes its functionality more explicit. Type hints do not make Python a statically typed language, and they do not affect its performance in any way, but together with a third party static analysis tool, they can create a powerful instrument that can benefit software development, especially when working on large, complex projects.
I also recommend watching this presentation by Guido van Rossum, where he explains the motivation and functionality of this feature.