Swift Functional Programming – Cheat Sheet
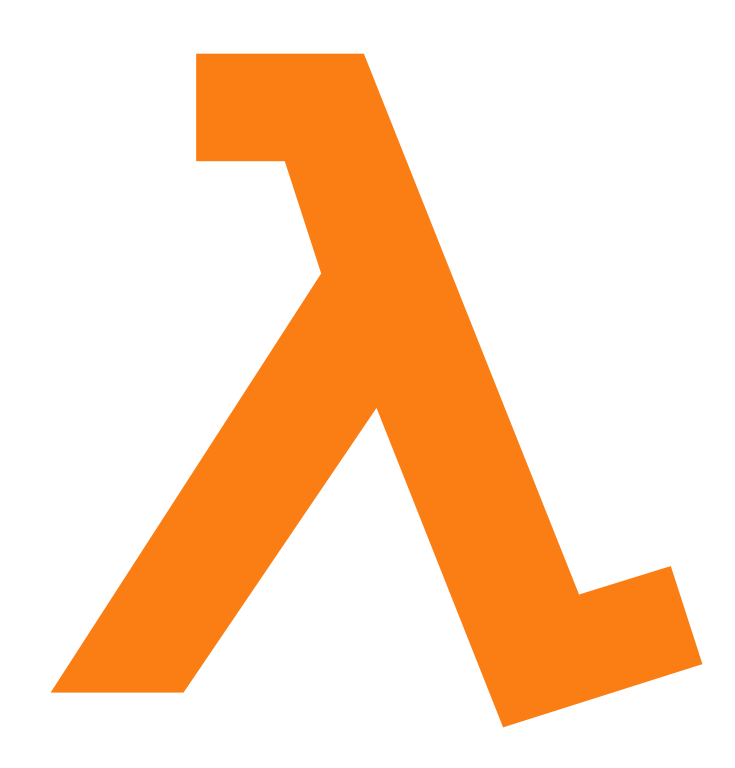
Functional programming has become very popular recently, it simplifies many aspects of development, however it’s not so easy to understand its principles. Even for those who understand how to switch to functional programming, it can be hard to start using it in real projects. To help, we prepared a cheat sheet, which can be printed and stuck next to your monitor to remind you about some of the most basic ways to make your code more functional.
Immutability – property, or data that is not allowed to change over the course of a program.
//wrong: var x = 1 x=2 //good: let one = 1 let two = one + 1
Modularity – each method should be responsible for one strict operation.
//wrong: func processData() { //fetch //sort //print } //good: func fetchData() -> Data { //fetch } func sortData(data:Data) -> Data { //sort } func printData(data:Data) { //print }
Functions are first-class citizens – functions can be assigned to values and treated like any other variables.
func numberIsEven(number: Int) -> Bool { return number%2 == 0 } let numbers = [1,2,3,4,5,6] let evenNumbers = numbers.filter(numberIsEven) //2,4,6
Partial Functions – allows you to encapsulate one function within another.
func isMultipleOf(n: Int, i: Int) -> Bool { return i % n == 0 } let r = 1...10 let evens = r.filter({ isMultipleOf(n: 2, i: $0) })
Pure Functions – functions always produces the same output when given the same input and create zero side effects outside of it.
func numbersLowerThan(_ number: Int, from numbers: [Int]) -> [Int] { return numbers.filter { $0 < number } } numbersLowerThan(3, from: [1,2,3,4])
Referential Transparency – element of a program is referentially transparent if you can replace it with its definition and always produce the same result. Pure functions satisfy this condition.
Recursion – occurs whenever a function calls itself as part of its function body.
func countTo(_ number: Int) { if number > 1 { countTo(number - 1) } print(number) }