How to test an open-source Django app
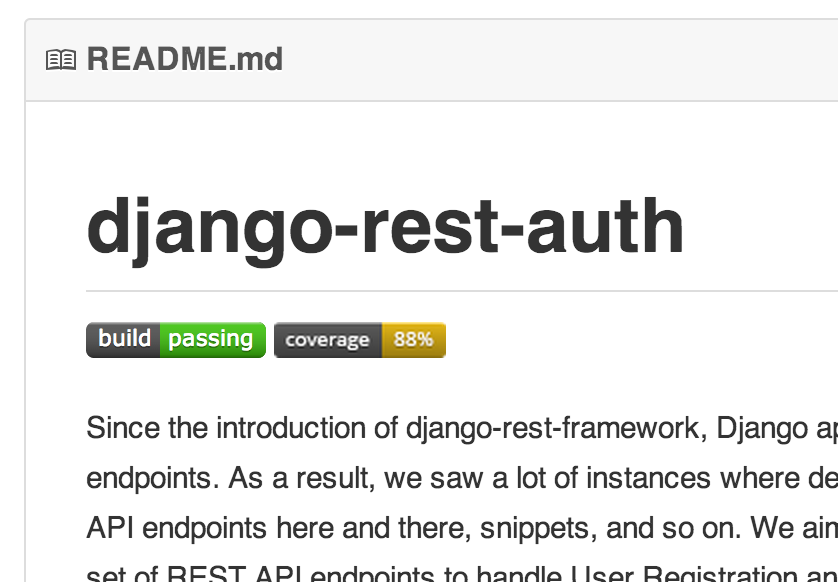
A few days ago, Tivix released a new open-source project named django-rest-auth – it's a Django application that exposes RESTful APIs for registration and authentication. It's super easy to use for single-page apps or mobile apps (you can read more about that in Silin's post).
Because I’m one of the contributors I wanted to make this project more reliable and encourage other developers to contribute, so I decided to write unit tests, integrate it with a continuous integration server, and measure test coverage.
I found two services that are very helpful with this process. Best of all, they are both are free for open source projects:
- Travis CI is a hosted continuous integration service. It is integrated with GitHub and offers first class support for many of languages, like Python, Ruby, PHP, Java, C/C++, etc. You can easily test your project against one or more versions of a language and even data stores.
- Coveralls works with your continuous integration server to give you test coverage history and statistics
Here is a quick tutorial how to integrate your open source Django app with Travis CI and Coveralls. All code examples are based on django-rest-auth project, so these links might be helpful: github repo, Travis CI, Coveralls
- Make your tests runnable from a standalone application
- Create django settings only for tests, let’s name it test_settings.py and put it inside application
- Add your custom test runner inside your app (runtests.py)
import os, sys os.environ['DJANGO_SETTINGS_MODULE'] = 'test_settings' test_dir = os.path.dirname(__file__) sys.path.insert(0, test_dir) from django.test.utils import get_runner from django.conf import settings def runtests(): TestRunner = get_runner(settings) test_runner = TestRunner(verbosity=1, interactive=True) failures = test_runner.run_tests(['your_app']) sys.exit(bool(failures))
- Define test_suite in your setup.py file with path to testrunner which we defined in previous step
test_suite='your_app.runtests.runtests'
- After that, your application should be testable by calling:
python setup.py test
- Sign up to Travis CI using your github account and activate github hook
After that you should be able to see your projects, activate application you want to test. - Create configuration file for Travis CI
- Create .travis.yml file in your project root. Notice that you can choose Python and Django versions to test against.
language: python python: - "2.6" - "2.7" env: - DJANGO=1.5.7 - DJANGO=1.6.4 install: - pip install -q Django==$DJANGO --use-mirrors script: - python setup.py test
- You can validate your config file before pushing it to repo by using travis-lint
- Make commit and push it to the repo, that will trigger test build on Travis CI. Here you can read more about getting started with Travis CI.
- Create .travis.yml file in your project root. Notice that you can choose Python and Django versions to test against.
- Coveralls – sign up using your github account and activate your project
- Integrate coveralls with application
- You will actually need to do three things in .travis.yml file: add coveralls app into requirements, change test command, call coveralls after success. Now, it should look like this:
language: python python: - "2.6" - "2.7" env: - DJANGO=1.5.7 - DJANGO=1.6.4 install: - pip install -q Django==$DJANGO --use-mirrors - pip install coveralls script: - coverage run --source=your_app setup.py test after_success: - coveralls
- Making a commit and pushing to the repo will trigger Travis test build and after successfully finish coverage data should be passed to Coveralls service. Now you should be able to see coverage scores on your dashboard.
- You will actually need to do three things in .travis.yml file: add coveralls app into requirements, change test command, call coveralls after success. Now, it should look like this:
- Puth badges in your readme file Both services support “readme badges,” so you can tell users that your tests are passing and what the test coverage score is.