Backend Development with Swift
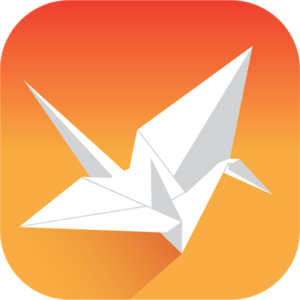
Why Swift?
Why chose Swift as a backend language? Have you ever dreamed about writing client and server in one language? Sure you did, but till now the most popular solution was JavaScript. Will Swift be the next one worth considering? I strongly believe so!
Before I show you how easily you can build a server in Swift, let me present some performance results, so you can be sure, that Swift is the right choice, performance-wise:
Picture Source: https://realm.io/news/tryswift-chris-robert-end-to-end-application-development-swift-backend/
Still not sure? Let’s compare memory footprint:
Picture Source: https://realm.io/news/tryswift-chris-robert-end-to-end-application-development-swift-backend/
As the above tests (performed by IBM) clearly indicate, Swift is well worth considering as the next programming language for backend. A lot of developers have also noticed that and currently there are three main frameworks (open sourced), which are making backend development easier with Swift. At Tivix we decided (based on our own tests and below benchmark) to use Perfect.
Picture Source: https://medium.com/@rymcol/linux-ubuntu-benchmarks-for-server-side-swift-vs-node-js-db52b9f8270b#.x18fah265
Hello Tivix!
The perfect starting point for your first backend app written in Swift is a template provided by Perfect. This template contains a project configured and ready to run immediately after download. The project requires xCode 8.0 and Swift 3.0. See below for the proper structure of the opened project:
In the case of a simple app, all code can be stored in main.swift class, which is the entry point. And by making a few simple changes, we are able to put logic taken from the mobile iOS app into a new server:
import PerfectLib import PerfectHTTP import PerfectHTTPServer import Foundation // Create HTTP server. let server = HTTPServer() // Create the container variable for routes to be added to. var routes = Routes() // Register your own routes and handlers routes.add(method: .get, uri: "/", handler: { request, response in // Setting the response content type explicitly to text/html response.setHeader(.contentType, value: "text/html") // Adding some HTML to the response body object let formatter = DateFormatter() formatter.dateFormat = "h:mm a 'on' MMMM dd, yyyy" let date = Date() formatter.timeZone = TimeZone(abbreviation: "PST") var content = "<br>Tivix San Francisco (formatter.string(from: date))" content += "<br>Tivix Portland (formatter.string(from: date))" formatter.timeZone = TimeZone(abbreviation: "EST") content += "<br>Tivix New York (formatter.string(from: date))" formatter.timeZone = TimeZone(abbreviation: "GMT") content += "<br>Tivix London (formatter.string(from: date))" formatter.timeZone = TimeZone(abbreviation: "CET") content += "<br>Tivix Poland (formatter.string(from: date))" response.appendBody(string: "<html><title>Tivix Time</title><body><h2>Tivix Time:</h2>(content)</body></html>") // Signalling that the request is completed response.completed() }) // Add the routes to the server. server.addRoutes(routes) server.serverPort = 8080 do { // Launch the HTTP server. try server.start() } catch PerfectError.networkError(let err, let msg) { print("Network error thrown: (err) (msg)") }
This app can be easily run from xCode. Using the above code will show you the local times in all Tivix offices around the world!
One last, but important thing to note is that the Perfect framework currently supports deployment directly to Heroku, or any other server using Docker.