Practical Coding
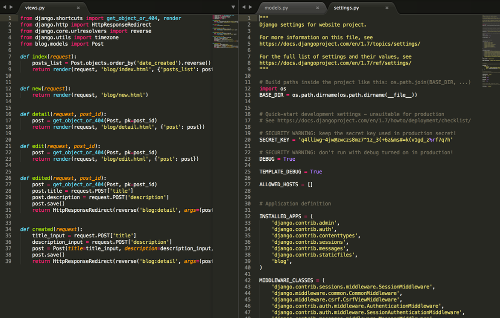
Recently, while working on improving my knowledge of common software engineering practices, I picked up two books: Pragmatic Programmer by Andrew Hunt and David Thomas and Code Complete 2 by Steve McConnell. I found both of these books to give excellent insight into overall software development practices that are, for the most part, language- and industry-independent.
While reading, I made notes of things relevant to my current occupation in web development, specifically with Python/Django. While Django as a framework provides a solid foundation, with many pre-existing standards and practices, developers still must maintain solid architecture of the application.
Below is the list of a few common practices which, in my opinion, can significantly improve software quality and efficiency.
- Separation of concerns: Although a programmer is free to put business and presentation logic into every module within a Django app, it’s best to follow Django convention and separate logic accordingly. Views should contain the presentation logic, while business logic should go in Models. When a View or a Model start growing and turning into a “God Object,” it’s good practice to move some of its logic to a helper class or function.
- Follow the coding standards of the programming language, which define guidelines for naming, indentation, spacing and other things. The main standard for Python is PEP 8. Following the same style is very important, as it allows you to keep code more consistent and readable.
- Just like any language, frameworks have a set of standards as well. It’s important to follow Django docs recommendations on how to do things. Django has certain specifications for folder structure, naming conventions, recommendations for using various Django components and so on. It helps to maintain the same structure throughout the entire project.
- Follow similar logic across the app as much as possible. It can be a naming convention, common logic, etc. For example, if one model has a
.get_absolute_url()
method, it’s bad practice to call the same method in another model just.absolute_url()
. Another example: each model usually has a main human-readable identifier which can be called name, title or label. It can be very helpful to use the same variable name for such identifier across the app, or at least for similar models.
- Code changes at a very constant rate and it is really easy to miss the moment when you start violating the DRY (Don’t Repeat Yourself) principle. For this reason, it’s important to revisit certain parts of the app to see what can be refactored to abstract common functionality. Given that refactoring can be a time-consuming process, it is important to find a balance and learn to prioritize in order to figure out which parts need refactoring the most.
- Writing automated tests may not be the most pleasant aspect of software development, but it definitely pays off as the project’s complexity grows. Well-tested code gives confidence that everything works as expected when adding new features or upgrading a third-party library.
These are just few examples of common software practices applied to Django development. They can simplify the development and maintenance process but they are by no means enough. There are many other important rules, standards, and security practices that are essential for every Python/Django developer to know. A recommendation for further reading is Two Scoops of Django, by Daniel Roy Greenfeld and Audrey Roy Greenfeld. It has very good coverage of Django-specific development practices. Also, of course, Django docs.